[Jest] - partial mock function in module in Jest P2
Truong Bui
Published on:
•
Last updated:
Problem
Continuing from the previous post , the complexFormula function has now been moved to the math file.
File name: math.ts
export const add = (a: number, b: number) => a + b;
export const subtract = (a: number, b: number) => a - b;
export const multiply = (a: number, b: number) => a * b;
export const complexFormula = (a: number, b: number) => {
const sum = add(a, b);
const mul = multiply(a, sum);
return subtract(mul, b);
};
Solution
Before go to solution, I want illustrate the relationship between math module and add function.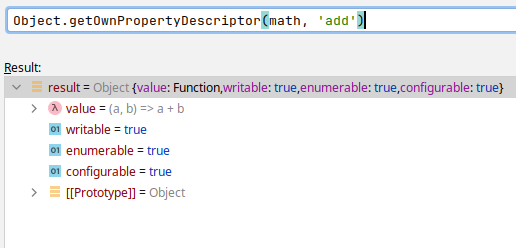
File name: math.test.ts
import * as math from './math';
const mockAddFn = jest.fn();
describe('complexFormula', () => {
beforeEach(() => {
Object.defineProperty(math, 'add', {
value: mockAddFn,
});
});
it('should return correct value', () => {
mockAddFn.mockReturnValue(10);
const result = math.complexFormula(1, 2);
expect(result).toBe(8);
});
});
File name: math.test.ts
import * as math from './math';
const mockAddFn = jest.fn();
describe('complexFormula', () => {
beforeEach(() => {
(math as any).add = mockAddFn; // we need to cast to any because add is readonly
});
it('should return correct value', () => {
mockAddFn.mockReturnValue(10);
const result = math.complexFormula(1, 2);
expect(result).toBe(8);
});
});
Hope you find this article useful. If you have any questions, please let me know in the comment section below. 👇